2) Write a C-program to input two initials of your name, your height in inches and weigh stones; convert the height to centimetres and weight to kilograms and display the follow results. PERSONAL DETAILS AGRIC IDENTIFICATION: BH HEIGHT (cm): 180 WEIGHT (Kg): 75 Tote: 1 inch 2.54 cm and 1 stone \( =6.364 \mathrm{Kg} \) 3. Convert a temperature reading in degrees Fahrenheit to degrees Celsius, using the formul C = (5/9) * (F-32). Test the program with the following values: \( 68,150,212,0,-22,-200 \) (degrees Fahrenheit)
Solución de tutoría real
Responder
Solución

¡Inicia sesión para desbloquear respuestas gratis!
Una plataforma de aprendizaje en la que confían millones de estudiantes y profesores reales.

Revisado y aprobado por el equipo de tutoría de UpStudy
Extra Insights
Here's a simple C program that meets your requirements:
```c
#include
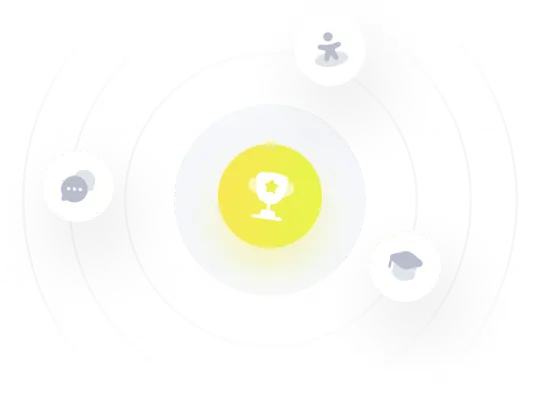