\( f(x)=x^{3}+x-1=0 \) Solve this by using bisection methes fixed poind and Mewton's Method. code this with Matlab
Solución de tutoría real
Responder
Solución
Revisado y aprobado por el equipo de tutoría de UpStudy


Mind Expander
To solve the equation \( f(x) = x^3 + x - 1 = 0 \) using the bisection method, fixed-point iteration, and Newton's method, we can outline the methods and provide a sample MATLAB code. ### Bisection Method The bisection method works by narrowing down the interval where the function changes sign. You first need to identify an interval \([a, b]\) such that \(f(a)\) and \(f(b)\) have opposite signs. Then, repeatedly bisect the interval until you find the root. ### Fixed-Point Iteration In fixed-point iteration, you rewrite the equation in the form \(x = g(x)\) and then iteratively apply \(g\) until convergence. ### Newton's Method Newton’s method requires an initial guess and uses the derivative of the function to find the roots. You update your guess using the formula \( x_{n+1} = x_n - \frac{f(x_n)}{f'(x_n)} \). ### Sample MATLAB Code ```matlab function root_finding_methods() % Define the function and its derivative f = @(x) x^3 + x - 1; % Function df = @(x) 3*x^2 + 1; % Derivative % Bisection method a = 0; b = 1; % Interval [0, 1] tol = 1e-6; % Tolerance while (b - a) / 2 > tol c = (a + b) / 2; % Midpoint if f(c) == 0 % Found exact solution break; elseif f(a) * f(c) < 0 b = c; % Root in left half else a = c; % Root in right half end end root_bisection = (a + b) / 2; % Fixed-point iteration g = @(x) (1 - x^3)^(1/3); % g(x) = (1 - x^3)^(1/3) x0 = 0.5; % Initial guess tol_fixed = 1e-6; % Tolerance x_fixed = x0; for i = 1:1000 % Max iterations x_next = g(x_fixed); if abs(x_next - x_fixed) < tol_fixed break; end x_fixed = x_next; end root_fixed_point = x_fixed; % Newton's method x_newton = 0.5; % Initial guess tol_newton = 1e-6; % Tolerance for i = 1:1000 % Max iterations x_newton = x_newton - f(x_newton)/df(x_newton); if abs(f(x_newton)) < tol_newton break; end end root_newton = x_newton; % Display results fprintf('Bisection root: %f\n', root_bisection); fprintf('Fixed-point root: %f\n', root_fixed_point); fprintf('Newton root: %f\n', root_newton); end ``` Run this function in MATLAB to find the roots using the three methods. Each method allows you to see a different way of tackling the problem, illustrating the strengths and specific use cases of each approach. Have fun experimenting with different initial guesses and intervals!
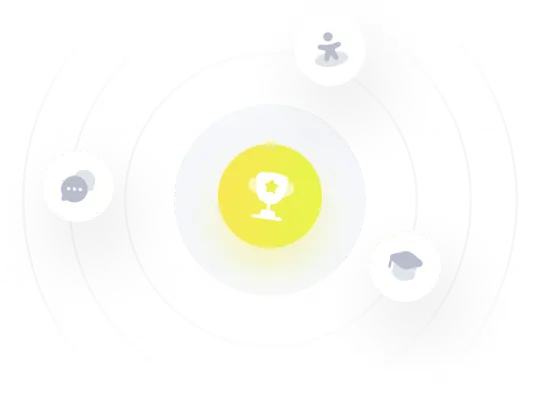